Python for loop
Python for loop can iterate over any sequence of items, such as a list or a string.
grammar:
The syntax for loop is as follows:
for iterating_var in sequence: statements(s)
flow chart:
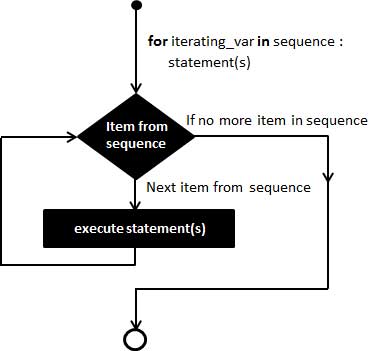
Example:
#!/usr/bin/python # -*- coding: UTF-8 -*- for letter in 'Python': # 第一个实例 print '当前字母 :', letter fruits = ['banana', 'apple', 'mango'] for fruit in fruits: # 第二个实例 print '当前字母 :', fruit print "Good bye!"
Examples of the above output:
当前字母 : P 当前字母 : y 当前字母 : t 当前字母 : h 当前字母 : o 当前字母 : n 当前字母 : banana 当前字母 : apple 当前字母 : mango Good bye!
By iterative sequence index
Another way is to execute the loop traverses through the index, the following examples:
#!/usr/bin/python # -*- coding: UTF-8 -*- fruits = ['banana', 'apple', 'mango'] for index in range(len(fruits)): print '当前水果 :', fruits[index] print "Good bye!"
Examples of the above output:
当前水果 : banana 当前水果 : apple 当前水果 : mango Good bye!
The above examples we use the built-in function len () and range (), len () function returns the length of the list, that is the number of elements. range Returns the number of a sequence.
Recycled else statements
In python, for ... else expressed so mean, for statements and ordinary no difference, else the statement is executed in the case of the normal cycle of execution End (ie for not interrupted by the break out of the) of, while ... else is the same.
The following examples:
#!/usr/bin/python # -*- coding: UTF-8 -*- for num in range(10,20): # 迭代 10 到 20 之间的数字 for i in range(2,num): # 根据因子迭代 if num%i == 0: # 确定第一个因子 j=num/i # 计算第二个因子 print '%d 等于 %d * %d' % (num,i,j) break # 跳出当前循环 else: # 循环的 else 部分 print num, '是一个质数'
Examples of the above output:
10 等于 2 * 5 11 是一个质数 12 等于 2 * 6 13 是一个质数 14 等于 2 * 7 15 等于 3 * 5 16 等于 2 * 8 17 是一个质数 18 等于 2 * 9 19 是一个质数