C # Array (Array)
Arrays are stored in a sequence of fixed-size collection of elements of the same type. An array is used to store a collection of data, usually considered an array is a collection of variables of the same type.
Declaration of an array variable is not declared number0, number1, ..., number99 a separate variable, but a declaration like this variable numbers, then use the numbers [0], numbers [1], ..., numbers [ 99] to represent a separate variable. An array element specified by the index is accessed.
All arrays are made of contiguous memory locations thereof. Lowest address corresponds to the first element, the highest address corresponding to the last element.
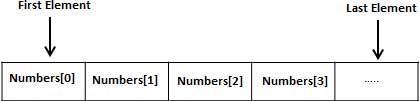
Declare an array
Declare an array in C #, you can use the following syntax:
datatype [] arrayName;
among them,
- datatypeis used to specify the type of the elements stored in the array.
- []Specified array rank (dimension). Rank specify the size of the array.
- arrayNamespecify the name of the array.
E.g:
double [] balance;
Array initialization
Declare an array does not initialize an array in memory. When initializing an array variable, you can assign to the array.
An array is a reference type, so you need to use thenew keyword to create an instance of the array.
E.g:
double [] balance = new double [10];
Assigned to the array
You can be assigned to a single array element by using the index number, for example:
double [] balance = new double [10]; balance [0] = 4500.0;
You can declare an array to array assignment at the same time, such as:
double [] balance = {2340.0, 4523.69, 3421.0};
You can also create and initialize an array, such as:
int [] marks = new int [5] {99, 98, 92, 97, 95};
In the above case, you can omit the size of the array, for example:
int [] marks = new int [] {99, 98, 92, 97, 95};
You can also assign an array variable to another array variable target. In this case, the source and the target will point to the same memory location:
int [] marks = new int [] {99, 98, 92, 97, 95}; int [] score = marks;
When you create an array, C # compiler will implicitly initialized array type of each array element to a default value. For example, all the elements int array will be initialized to 0.
Access array elements
Elements are indexed by name to access the array. This is done by placing the index of the element in square brackets after the name of the array to achieve. E.g:
double salary = balance [9];
Here is an example using the three concepts mentioned above, that statement, assignments, access the array:
using System; namespace ArrayApplication { class MyArray { static void Main (string [] args) { int [] n = new int [10]; / * n is an integer array with 10 * / int i, j; / * Initialize an array of n elements * / for (i = 0; i <10; i ++) { n [i] = i + 100; } / * The value of the output of each array element * / for (j = 0; j <10; j ++) { Console.WriteLine ( "Element [{0}] = {1}", j, n [j]); } Console.ReadKey (); } } }
When the above code is compiled and executed, it produces the following results:
Element [0] = 100 Element [1] = 101 Element [2] = 102 Element [3] = 103 Element [4] = 104 Element [5] = 105 Element [6] = 106 Element [7] = 107 Element [8] = 108 Element [9] = 109
Useforeachloop
In the previous example, we use a for loop to access each array element. You can also use aforeach statement to traverse an array.
using System; namespace ArrayApplication { class MyArray { static void Main (string [] args) { int [] n = new int [10]; / * n is an integer array with 10 * / / * Initialize an array of n elements * / for (int i = 0; i <10; i ++) { n [i] = i + 100; } / * The value of the output of each array element * / foreach (int j in n) { int i = j-100; Console.WriteLine ( "Element [{0}] = {1}", i, j); } Console.ReadKey (); } } }
When the above code is compiled and executed, it produces the following results:
Element[0] = 100 Element[1] = 101 Element[2] = 102 Element[3] = 103 Element[4] = 104 Element[5] = 105 Element[6] = 106 Element[7] = 107 Element[8] = 108 Element[9] = 109
C # Array Details
In C #, arrays are very important and need to know more details. The following lists the C # programmer must be aware of some important concepts related to the array:
概念 | 描述 |
---|---|
多维数组 | C# 支持多维数组。多维数组最简单的形式是二维数组。 |
交错数组 | C# 支持交错数组,即数组的数组。 |
传递数组给函数 | 您可以通过指定不带索引的数组名称来给函数传递一个指向数组的指针。 |
参数数组 | 这通常用于传递未知数量的参数给函数。 |
Array 类 | 在 System 命名空间中定义,是所有数组的基类,并提供了各种用于数组的属性和方法。 |