Node.js module system
To make Node.js file can call each other, Node.js provides a simple modular system.
Module is an essential part of Node.js applications, files and modules is one to one. In other words, a Node.js file is a module, this file may be JavaScript code, JSON or compiled C / C ++ extensions.
Create a module
In Node.js, create a module is very simple, as we create a 'main.js' file, as follows:
var hello = require('./hello'); hello.world();
The above example, the code require ( './ hello') introduced the current hello.js file directory (./ current directory, node.js default suffix js).
Node.js provides exports and require two objects, where exports are open module interfaces require a module for acquiring from the external interface, namely the exports target acquisition module.
Next we come to create hello.js file, as follows:
exports.world = function() { console.log('Hello World'); }
In the example above, hello.js exports by the world as an object access interface module, this module in main.js loaded via require ( './ hello'), then you can directly access the object's members hello.js in exports the function.
Sometimes we just want to package an object module, the format is as follows:
module.exports = function() { // ... }
E.g:
//hello.js function Hello() { var name; this.setName = function(thyName) { name = thyName; }; this.sayHello = function() { console.log('Hello ' + name); }; }; module.exports = Hello;
So you get a direct object:
//main.js var Hello = require('./hello'); hello = new Hello(); hello.setName('BYVoid'); hello.sayHello();
The only change is the use of an interface module module.exports = Hello replaced exports.world = function () {}. When referencing the module outside, Hello object whose interface object is to be output itself, rather than the original exports.
Where to put the server module
Perhaps you have noticed that we have used in the module code. like this:
var http = require("http"); ... http.createServer(...);
Node.js comes with a feature called "http" module, we ask it to return value to a local variable in our code.
This brings us into a local variable has all the public methods the http module provides an object.
Node.js methods require the file search strategy is as follows:
Since there are four class module (primary module and three modules file) Node.js, although require method is extremely simple, but inside it is very complex loading, load their priorities are different. As shown below:
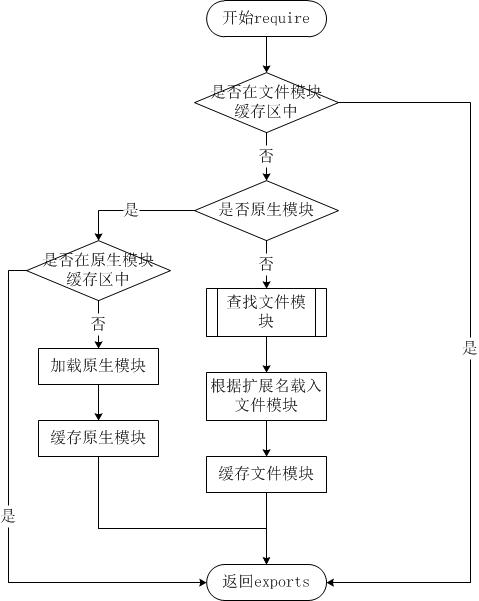
Loaded from the file cache module
Despite the different priorities native module and file module, but it will not take precedence over existing load module file from the cache module.
Loading from native module
Native module priority after the priority file cache module. require methods After parsing the file name, priority check whether the module in a native module list. In http module, for example, although there is a http / http.js / http.node / http.json file in the directory, require ( "http") will not be loaded from the file, but loaded from the native module.
Native module also has a buffer zone, it is also preferentially loaded from the cache. If the buffer is not loaded before, then call the native module loading will be loaded and executed.
Load from file
When the file does not exist in the cache module, and not a native module when, Node.js will require analytical method incoming parameters, and load the actual file from the file system, the loading process of compiling and packaging details in the previous section It has been introduced, where we will describe in detail the process to find the file module, which also has some details worth knowing.
require transfer method accepts the following parameters:
- http, fs, path, etc., native module.
- ./mod or ../mod, relative path file module.
- / Pathtomodule / mod, the absolute path of the file module.
- mod, non-native module file module.