Service Locator pattern
Service Locator pattern (Service Locator Pattern) for use in positioning we want to use JNDI lookup services when. Taking into account the JNDI lookup high price for a service, the Service Locator pattern full use of caching technology. In the first request for a service, the service in the JNDI lookup service locator, and caches the service object. When requesting the same service again, the service locator looks in its cache, which can improve application performance to a large extent. The following is an entity of this design pattern.
- Service (Service) - services actually handling the request.References to these services can be found at JNDI server.
- Context / Initial Context - JNDI Context with a service you want to find a reference.
- The service locator (Service Locator) - service locator is through JNDI lookup and caching services to get a single point of contact service.
- Cache (Cache) - a reference to the cache storage services, in order to reuse them.
- Client (Client) - Client object invocation of the service through ServiceLocator.
achieve
We will createServiceLocator,InitialContext, Cache, Service as an entity represents various objects.Service1andService2represent entity services.
ServiceLocatorPatternDemo,our demo class here as a client, will be used to demonstrateServiceLocatorservice locator design pattern.
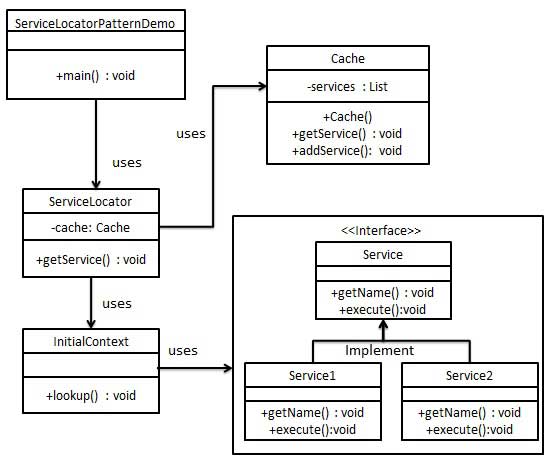
step 1
Create a service interface Service.
Service.java
public interface Service { public String getName (); public void execute (); }
Step 2
Create a service entity.
Service1.java
public class Service1 implements Service { public void execute () { System.out.println ( "Executing Service1"); } @Override public String getName () { return "Service1"; } }
Service2.java
public class Service2 implements Service { public void execute () { System.out.println ( "Executing Service2"); } @Override public String getName () { return "Service2"; } }
Step 3
Create a query for the JNDI InitialContext.
InitialContext.java
public class InitialContext { public Object lookup (String jndiName) { if (jndiName.equalsIgnoreCase ( "SERVICE1")) { System.out.println ( "Looking up and creating a new Service1 object"); return new Service1 (); } Else if (jndiName.equalsIgnoreCase ( "SERVICE2")) { System.out.println ( "Looking up and creating a new Service2 object"); return new Service2 (); } return null; } }
Step 4
Create Cache Cache.
Cache.java
import java.util.ArrayList; import java.util.List; public class Cache { private List <Service> services; public Cache () { services = new ArrayList <Service> (); } public Service getService (String serviceName) { for (Service service: services) { if (service.getName (). equalsIgnoreCase (serviceName)) { System.out.println ( "Returning cached" + serviceName + "object"); return service; } } return null; } public void addService (Service newService) { boolean exists = false; for (Service service: services) { if (service.getName (). equalsIgnoreCase (newService.getName ())) { exists = true; } } if (! exists) { services.add (newService); } } }
Step 5
Create a service locator.
ServiceLocator.java
public class ServiceLocator { private static Cache cache; static { cache = new Cache (); } public static Service getService (String jndiName) { Service service = cache.getService (jndiName); if (service! = null) { return service; } InitialContext context = new InitialContext (); Service service1 = (Service) context.lookup (jndiName); cache.addService (service1); return service1; } }
Step 6
UseServiceLocatorto demonstrate the service locator design pattern.
ServiceLocatorPatternDemo.java
public class ServiceLocatorPatternDemo { public static void main (String [] args) { Service service = ServiceLocator.getService ( "Service1"); service.execute (); service = ServiceLocator.getService ( "Service2"); service.execute (); service = ServiceLocator.getService ( "Service1"); service.execute (); service = ServiceLocator.getService ( "Service2"); service.execute (); } }
Step 7
Verify output.
Looking up and creating a new Service1 object Executing Service1 Looking up and creating a new Service2 object Executing Service2 Returning cached Service1 object Executing Service1 Returning cached Service2 object Executing Service2