Visitor Pattern
In the visitor pattern (Visitor Pattern), we use a visitor class, it changes the execution algorithm element classes. In this way, with the implementation of the algorithm can be a visitor element is changed. This type of design patterns belong behavioral patterns. According pattern, element object has accepted the target visitors, so visitors can handle objects operating element object.
Introduction
Intent: The main structure of the data and data manipulation separation.
Mainly to solve: a stable data structure and variable operating coupling problem.
When to use: the need for a target structure of objects in many different and unrelated actions, but these actions need to avoid "pollution" of the class of these objects, the use of visitors to these packages to the class.
How to fix: the class is accessed inside plus a reception of foreign visitors to provide interface.
The key code: the data base class which has a method to accept visitors, itself a reference to incoming visitors.
Application Example: You are a guest at a friend's house, you are a visitor, a friend accepts your visit through your friend's description, then the description of a friend to make a judgment, which is the visitor pattern.
Advantages: 1, in line with the single responsibility principle.2, excellent scalability. 3, flexibility.
Disadvantages: 1, the specific elements of the visitor announced details of a violation of the principle of Demeter.2, the specific elements of change more difficult. 3, in violation of the Dependency Inversion principle, rely on a specific class that does not rely on abstraction.
Usage scenarios: 1, the object corresponding to the object class structure rarely changes, but often need to define a new operation on this object structure.2, the need for a target structure of objects in many different and unrelated actions, but these actions need to avoid "pollution" of the class of these objects, we do not want to modify these classes when you add a new operation.
Note: Visitors can be unified function, you can do a report, UI, intercept and filter.
achieve
We will create a definition of acceptable operationComputerPartinterface.Keyboard,Mouse, Monitor andComputerachieve the entity classesComputerPartinterface. We will define another interfaceComputerPartVisitor,which defines the type of operation to visitors.Computeruse Entity visitors to take appropriate action.
VisitorPatternDemo,we demonstrate the use of classComputer,ComputerPartVisitor class to demonstrate the use of the visitor pattern.
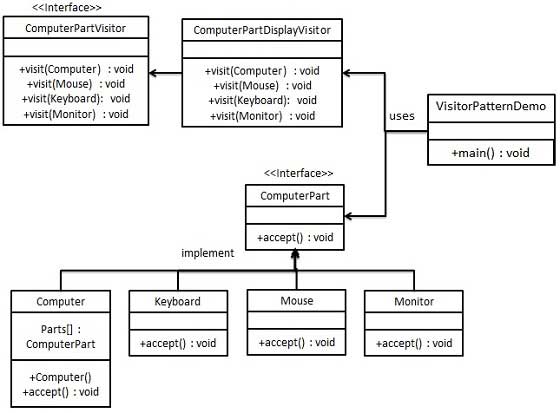
step 1
Define an interface that represents the element.
ComputerPart.java
public interface class ComputerPart { public void accept (ComputerPartVisitor computerPartVisitor); }
Step 2
Create extends the entity classes above class.
Keyboard.java
public class Keyboard implements ComputerPart { @Override public void accept (ComputerPartVisitor computerPartVisitor) { computerPartVisitor.visit (this); } }
Monitor.java
public class Monitor implements ComputerPart { @Override public void accept (ComputerPartVisitor computerPartVisitor) { computerPartVisitor.visit (this); } }
Mouse.java
public class Mouse implements ComputerPart { @Override public void accept (ComputerPartVisitor computerPartVisitor) { computerPartVisitor.visit (this); } }
Computer.java
public class Computer implements ComputerPart { ComputerPart [] parts; public Computer () { parts = new ComputerPart [] {new Mouse (), new Keyboard (), new Monitor ()}; } @Override public void accept (ComputerPartVisitor computerPartVisitor) { for (int i = 0; i <parts.length; i ++) { parts [i] .accept (computerPartVisitor); } computerPartVisitor.visit (this); } }
Step 3
Define a representation visitor interface.
ComputerPartVisitor.java
public interface ComputerPartVisitor { public void visit (Computer computer); public void visit (Mouse mouse); public void visit (Keyboard keyboard); public void visit (Monitor monitor); }
Step 4
Visitors entity created to achieve the above-mentioned class.
ComputerPartDisplayVisitor.java
public class ComputerPartDisplayVisitor implements ComputerPartVisitor { @Override public void visit (Computer computer) { System.out.println ( "Displaying Computer."); } @Override public void visit (Mouse mouse) { System.out.println ( "Displaying Mouse."); } @Override public void visit (Keyboard keyboard) { System.out.println ( "Displaying Keyboard."); } @Override public void visit (Monitor monitor) { System.out.println ( "Displaying Monitor."); } }
Step 5
UseComputerPartDisplayVisitorto show part of theComputer.
VisitorPatternDemo.java
public class VisitorPatternDemo { public static void main (String [] args) { ComputerPart computer = new Computer (); computer.accept (new ComputerPartDisplayVisitor ()); } }
Step 6
Verify output.
Displaying Mouse. Displaying Keyboard. Displaying Monitor. Displaying Computer.