Abstract factory pattern
Abstract Factory (Abstract Factory Pattern) is created around a super plant other plants. The plant is also known as super other factories factory. This type of design patterns belong create schema, which provides the best way to create objects.
In the abstract factory pattern, the interface is responsible for creating a plant related objects, without explicitly specifying their classes. Each generated object, according to the factory can provide factory model.
Introduction
Intent: to create a series of related or dependent objects interface, without specifying their concrete classes.
Mainly to solve: The main problem of the interface options.
When to use: System products have more than one product family, and the only system in which a family of consumer products.
How to fix: in a product family inside, define multiple products.
The key code: aggregate multiple similar products in a factory.
Application examples: work, in order to take part in some meetings, there must be two or more sets of clothes it, say, business attire (complete sets, a series of specific products), fashion dress (complete sets, a series of specific products), even for a family You may have a business women, business men's, women's fashion, men's fashion, they are also complete sets, namely a series of specific products.Suppose a situation (in reality does not exist, or else, not communism, but in favor of the abstract factory pattern description), in your home, one wardrobe (concrete factory) can only store a one such clothes (complete sets, a series of specific product), every time you take this set of clothes from the closet naturally taken out. With OO thinking to understand, all the wardrobe (concrete plant) are wardrobes class (abstract factory) one, and each one set of clothes and including specific jacket (a particular product), pants (a specific product), these are in fact specific shirt jacket (abstract products), specific pants are pants (another abstract product).
Pros: When a product family more objects are designed to work together, we can ensure that the client always use just the same product family objects.
Disadvantages: Extended product family is very difficult, to increase a range of a product, both in the abstract Creator Riga code, but also to add a specific code inside.
Usage scenarios: 1, QQ for the skin, a set of change together.2, generate a different operating system procedures.
Note: The extended product family is difficult, easy to expand the product level.
achieve
We will create theShapeandColorinterface and implementation class interface of these entities. The next step is to create an abstract factory classAbstractFactory.Then define the factory classShapeFactoryandColorFactory,these two classes are factory expandedAbstractFactory.Then create a factory creator / builder classFactoryProducer.
AbstractFactoryPatternDemo,our demonstration classes usingFactoryProducerto getAbstractFactoryobject. It passes shape informationShape(CIRCLE / RECTANGLE / SQUARE) toAbstractFactory,it needs to get the type of object. It also passes color informationColor(RED / GREEN / BLUE) toAbstractFactory,it needs to get the type of object.
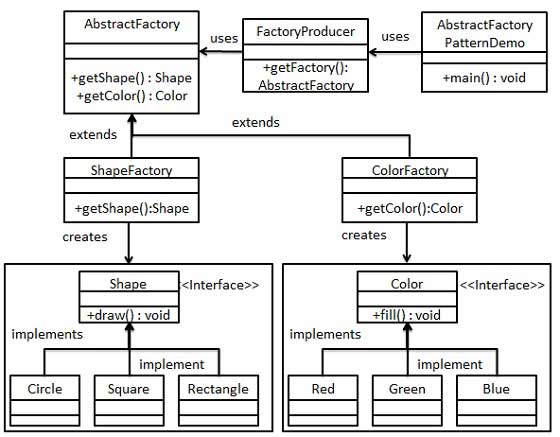
step 1
Interface to create a shape.
Shape.java
public interface Shape { void draw (); }
Step 2
Create entity class that implements the interface.
Rectangle.java
public class Rectangle implements Shape { @Override public void draw () { System.out.println ( "Inside Rectangle :: draw () method."); } }
Square.java
public class Square implements Shape { @Override public void draw () { System.out.println ( "Inside Square :: draw () method."); } }
Circle.java
public class Circle implements Shape { @Override public void draw () { System.out.println ( "Inside Circle :: draw () method."); } }
Step 3
Create an interface for color.
Color.java
public interface Color { void fill (); }
Step 4
Create entity class that implements the interface.
Red.java
public class Red implements Color { @Override public void fill () { System.out.println ( "Inside Red :: fill () method."); } }
Green.java
public class Green implements Color { @Override public void fill () { System.out.println ( "Inside Green :: fill () method."); } }
Blue.java
public class Blue implements Color { @Override public void fill () { System.out.println ( "Inside Blue :: fill () method."); } }
Step 5
Create an abstract class for the Color and Shape objects to get the factory.
AbstractFactory.java
public abstract class AbstractFactory { abstract Color getColor (String color); abstract Shape getShape (String shape); }
Step 6
Create extended AbstractFactory factory class, entity class object generation based on the given information.
ShapeFactory.java
public class ShapeFactory extends AbstractFactory { @Override public Shape getShape (String shapeType) { if (shapeType == null) { return null; } if (shapeType.equalsIgnoreCase ( "CIRCLE")) { return new Circle (); } Else if (shapeType.equalsIgnoreCase ( "RECTANGLE")) { return new Rectangle (); } Else if (shapeType.equalsIgnoreCase ( "SQUARE")) { return new Square (); } return null; } @Override Color getColor (String color) { return null; } }
ColorFactory.java
public class ColorFactory extends AbstractFactory { @Override public Shape getShape (String shapeType) { return null; } @Override Color getColor (String color) { if (color == null) { return null; } if (color.equalsIgnoreCase ( "RED")) { return new Red (); } Else if (color.equalsIgnoreCase ( "GREEN")) { return new Green (); } Else if (color.equalsIgnoreCase ( "BLUE")) { return new Blue (); } return null; } }
Step 7
Create a factory creator / builder category, passing through shape or color information to obtain the factory.
FactoryProducer.java
public class FactoryProducer { public static AbstractFactory getFactory (String choice) { if (choice.equalsIgnoreCase ( "SHAPE")) { return new ShapeFactory (); } Else if (choice.equalsIgnoreCase ( "COLOR")) { return new ColorFactory (); } return null; } }
Step 8
Use FactoryProducer to get AbstractFactory, to obtain the object of the class by passing the entity type information.
AbstractFactoryPatternDemo.java
public class AbstractFactoryPatternDemo { public static void main (String [] args) { // Get the factory shape AbstractFactory shapeFactory = FactoryProducer.getFactory ( "SHAPE"); // Get object shape Circle Shape shape1 = shapeFactory.getShape ( "CIRCLE"); // Call the draw method of shape1.draw Circle (); // Get object shape Rectangle Shape shape2 = shapeFactory.getShape ( "RECTANGLE"); // Call the draw method of shape2.draw Rectangle (); // Get object shape Square Shape shape3 = shapeFactory.getShape ( "SQUARE"); // Call the draw method Square shape3.draw (); // Get color Factories AbstractFactory colorFactory = FactoryProducer.getFactory ( "COLOR"); // Get the object color is Red Color color1 = colorFactory.getColor ( "RED"); // Call the fill method Red color1.fill (); // Get object color Green Color color2 = colorFactory.getColor ( "Green"); // Call Green's fill method color2.fill (); // Get object color Blue Color color3 = colorFactory.getColor ( "BLUE"); // Call the fill method Blue color3.fill (); } }
Step 9
Verify output.
Inside Circle :: draw () method. Inside Rectangle :: draw () method. Inside Square :: draw () method. Inside Red :: fill () method. Inside Green :: fill () method. Inside Blue :: fill () method.